はじめに
AWS BedrockのStable Diffusion XLで画像生成をやっていきます。こちらの記事を参考にしています。
導入
1.IAMロールを作成
2.アクセスキーとシークレットキーをメモ
3.ポリシーを作成
1 2 3 4 5 6 7 8 9 10 11 12 13 |
{ "Version": "2012-10-17", "Statement": [ { "Sid": "Statement1", "Effect": "Allow", "Action": [ "bedrock:*" ], "Resource": "*" } ] } |
4.AWS BedrockのModel access->EditからStable Diffusion XLにチェック
5.AWS CLIをインストール
6.コマンドプロンプトを開く
1 2 |
C:\Users\admin>aws --version aws-cli/2.13.30 Python/3.11.6 Windows/10 exe/AMD64 prompt/off |
7.aws configureでアクセスキーとシークレットキーを設定
1 2 3 4 5 |
C:\Users\admin>aws configure AWS Access Key ID [****************]: AWS Secret Access Key [****************]: Default region name [ap-northeast-1]: Default output format [None]: json |
8.anaconda promptを開き、Python 3.11環境を作成
1 2 |
(base) C:\Users\admin\Documents\PythonProjects>conda create -n py311 python=3.11 (base) C:\Users\admin\Documents\PythonProjects>conda activate py311 |
9.ライブラリのインストール
1 2 |
(py311) C:\Users\admin\Documents\PythonProjects>pip install --no-build-isolation --force-reinstall boto3>=1.28.57 awscli>=1.29.57 botocore>=1.31.57 (py311) C:\Users\admin\Documents\PythonProjects>pip install pillow==9.5 |
10.bedrockのツールをダウンロード
11.utilsをプロジェクトフォルダに置く

12.app.pyを作成。os.environ[“BEDROCK_ASSUME_ROLE”]はコメントアウトしてOK。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 |
# Python Built-Ins: import base64 import io import json import os import sys # External Dependencies: import boto3 from PIL import Image module_path = ".." sys.path.append(os.path.abspath(module_path)) from utils import bedrock, print_ww # ---- ⚠️ Un-comment and edit the below lines as needed for your AWS setup ⚠️ ---- os.environ["AWS_DEFAULT_REGION"] = "us-east-1" # E.g. "us-east-1" # os.environ["AWS_PROFILE"] = "<your_profile>" # os.environ["BEDROCK_ASSUME_ROLE"] = "arn:aws:iam::785208610362:user/s-fujimoto" # E.g. "arn:aws:iam::xxxxxxxxxxxx:role/xxxxxx" # E.g. boto3_bedrock = bedrock.get_bedrock_client( assumed_role=os.environ.get("BEDROCK_ASSUME_ROLE", None), region=os.environ.get("AWS_DEFAULT_REGION", None), ) print( "1 if the image is generated using a prompt, 2 if the image is generated using an image and a prompt." ) choice = int(input("Enter Choice :")) print(choice) if choice == 1: print("please enter the Prompt"), prompt = str(input()) print( "Please enter 3 negative Prompt eg:poorly rendered,poor background details,poorly drawn face" ) negative_prompts = [] n = 3 for i in range(0, n): # ele = [input(), str(input()),] ele = str(input()) negative_prompts.append(ele) print(negative_prompts) style_preset = "photographic" request = json.dumps( { "text_prompts": ( [{"text": prompt, "weight": 1.0}] + [ {"text": negprompt, "weight": -1.0} for negprompt in negative_prompts ] ), "cfg_scale": 5, "seed": 5450, "steps": 70, "style_preset": style_preset, } ) modelId = "stability.stable-diffusion-xl" response = boto3_bedrock.invoke_model(body=request, modelId=modelId) response_body = json.loads(response.get("body").read()) print(response_body["result"]) base_64_img_str = response_body["artifacts"][0].get("base64") print(f"{base_64_img_str[0:80]}...") os.makedirs("data", exist_ok=True) image_1 = Image.open( io.BytesIO(base64.decodebytes(bytes(base_64_img_str, "utf-8"))) ) image_1.save("data/image_1.png") image_1 if choice == 2: print("please enter the image file path"), image_path = str(input()) print("please enter the Prompt"), change_prompt = str(input()) print( "Please enter 3 negative Prompt eg:poorly rendered,poor background details,poorly drawn face" ) negative_prompts = [] n = 3 for i in range(0, n): # ele = [input(), str(input()),] ele = str(input()) negative_prompts.append(ele) print(negative_prompts) style_preset = "photographic" def image_to_base64(img) -> str: """Convert a PIL Image or local image file path to a base64 string for Amazon Bedrock""" if isinstance(img, str): if os.path.isfile(img): print(f"Reading image from file: {img}") with open(img, "rb") as f: return base64.b64encode(f.read()).decode("utf-8") else: raise FileNotFoundError(f"File {img} does not exist") elif isinstance(img, Image.Image): print("Converting PIL Image to base64 string") buffer = io.BytesIO() img.save(buffer, format="PNG") return base64.b64encode(buffer.getvalue()).decode("utf-8") else: raise ValueError(f"Expected str (filename) or PIL Image. Got {type(img)}") original_image = Image.open(image_path) image_1 = original_image.resize((512, 512)) init_image_b64 = image_to_base64(image_1) print(init_image_b64[:80] + "...") request = json.dumps( { "text_prompts": ( [{"text": change_prompt, "weight": 1.0}] + [ {"text": negprompt, "weight": -1.0} for negprompt in negative_prompts ] ), "cfg_scale": 10, "init_image": init_image_b64, "seed": 321, "start_schedule": 0.6, "steps": 50, "style_preset": style_preset, } ) modelId = "stability.stable-diffusion-xl" response = boto3_bedrock.invoke_model(body=request, modelId=modelId) response_body = json.loads(response.get("body").read()) print(response_body["result"]) image_2_b64_str = response_body["artifacts"][0].get("base64") print(f"{image_2_b64_str[0:80]}...") image_2 = Image.open( io.BytesIO(base64.decodebytes(bytes(image_2_b64_str, "utf-8"))) ) image_2.save("data/image.png") |
実行
オプション1は、プロンプトから画像生成します
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
(py311) C:\Users\admin\Documents\PythonProjects\Bedrocks>python app.py Create new client Using region: us-east-1 boto3 Bedrock client successfully created! bedrock-runtime(https://bedrock-runtime.us-east-1.amazonaws.com) 1 if the image is generated using a prompt, 2 if the image is generated using an image and a prompt. Enter Choice :1 1 please enter the Prompt japanese mountain Please enter 3 negative Prompt eg:poorly rendered,poor background details,poorly drawn face poorly rendered poor background details poorly drawn face ['poorly rendered', 'poor background details', 'poorly drawn face'] success iVBORw0KGgoAAAANSUhEUgAAAgAAAAIACAIAAAB7GkOtAAADWmVYSWZNTQAqAAAACAAGAQAABAAAAAEA... |
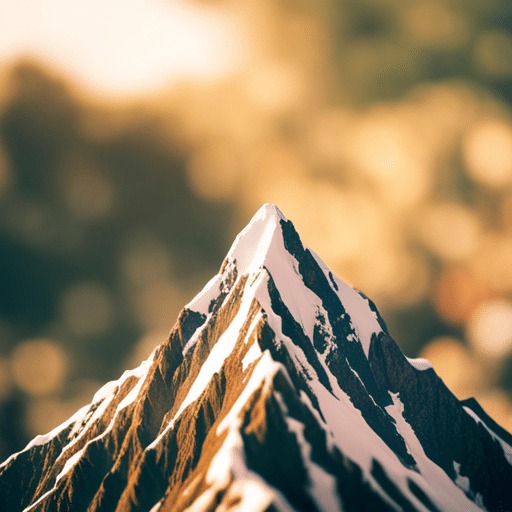
japanese mountain
オプションの2は、画像とプロンプトを入力して画像生成します
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
(py311) C:\Users\admin\Documents\PythonProjects\Bedrocks>python app.py Create new client Using region: us-east-1 boto3 Bedrock client successfully created! bedrock-runtime(https://bedrock-runtime.us-east-1.amazonaws.com) 1 if the image is generated using a prompt, 2 if the image is generated using an image and a prompt. Enter Choice :2 2 please enter the image file path image.jpg please enter the Prompt cute girl Please enter 3 negative Prompt eg:poorly rendered,poor background details,poorly drawn face poorly rendered poor background details poorly drawn face ['poorly rendered', 'poor background details', 'poorly drawn face'] Converting PIL Image to base64 string iVBORw0KGgoAAAANSUhEUgAAAgAAAAIACAIAAAB7GkOtAAABdmlDQ1BJQ0MgUHJvZmlsZQAAeJylkLFL... success iVBORw0KGgoAAAANSUhEUgAAAgAAAAIACAIAAAB7GkOtAACj0GVYSWZNTQAqAAAACAAGAQAABAAAAAEA... |
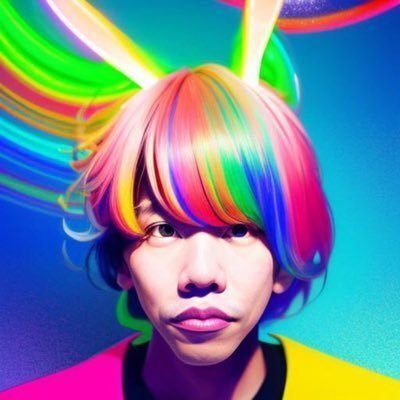
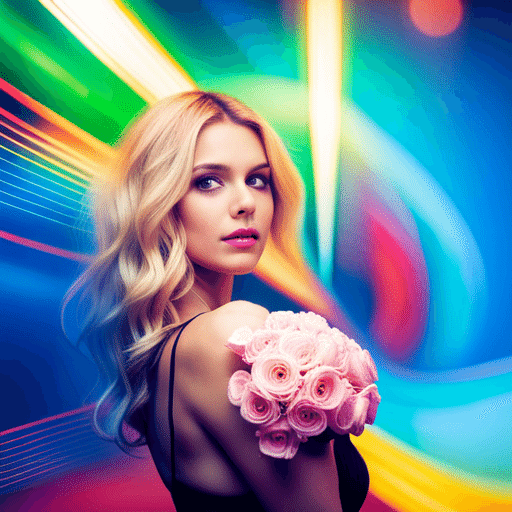
だいぶ変えてきたなw